Playwright is an open-source library developed by Microsoft that allows automating web browser tests. In addition to classic end-to-end tests, it offers advanced features to detect and analyze JavaScript errors that occur during test execution. As part of an audit I had to carry out, this feature proved extremely useful for identifying client-side bugs before they reach production.
Context
I have three HTML pages:
page0.html
, an HTML page with no errorspage1.html
, an HTML page generating a JS errorpage2.html
, an HTML page generating a JS warning
|
|
|
|
|
|
Setup
- Start by setting up the test environment using the command
npm init playwright@latest
- The command will ask a few questions about the setup:
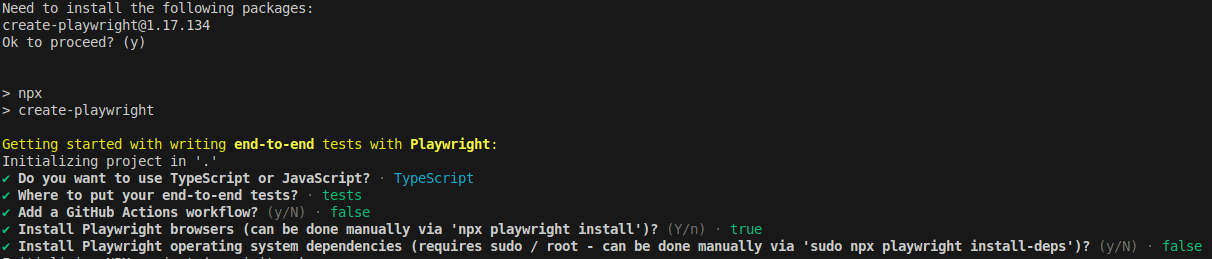
- The command will generate all the files and install the dependencies:
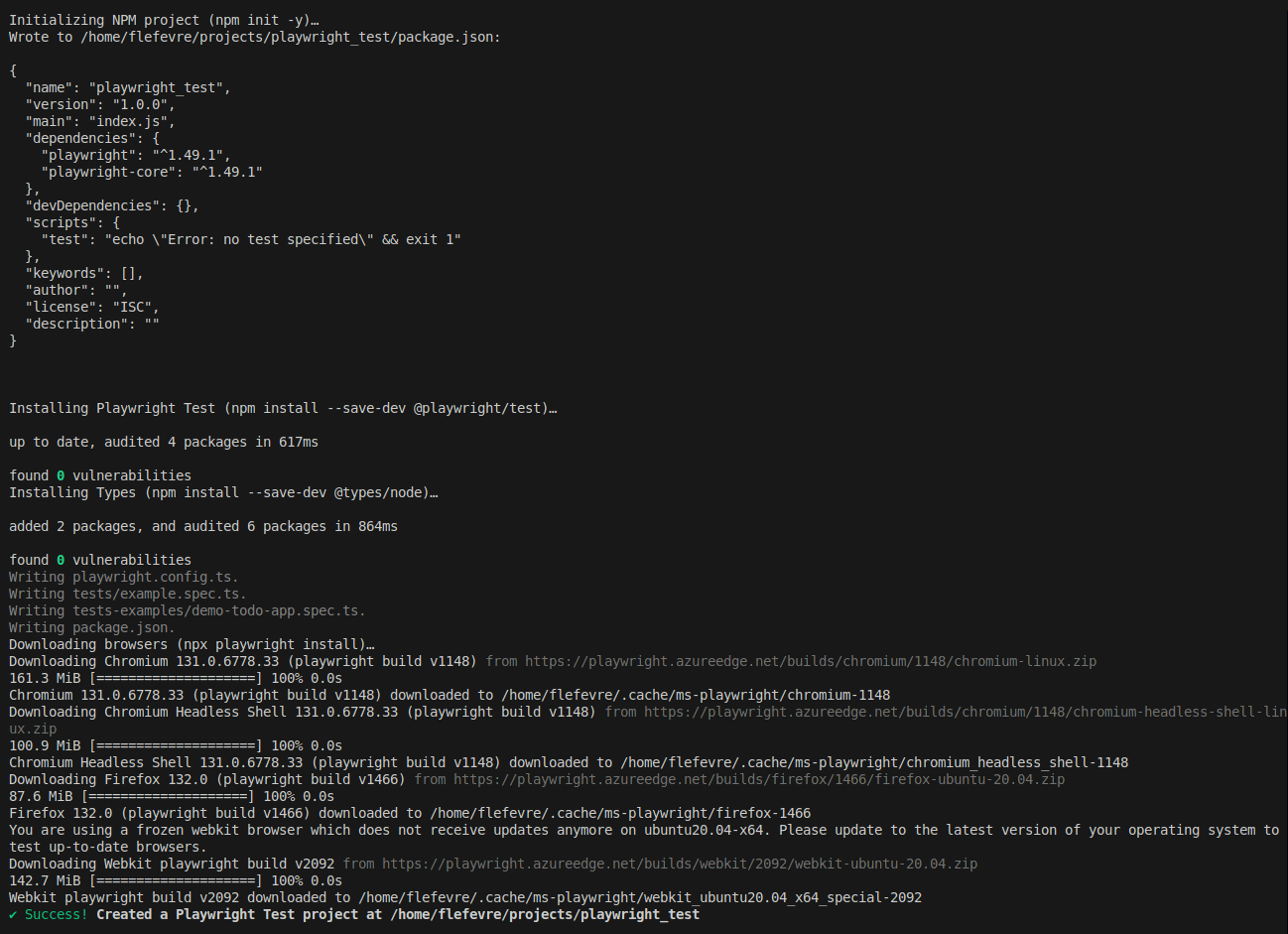
- The command will then provide some commands to use Playwright:
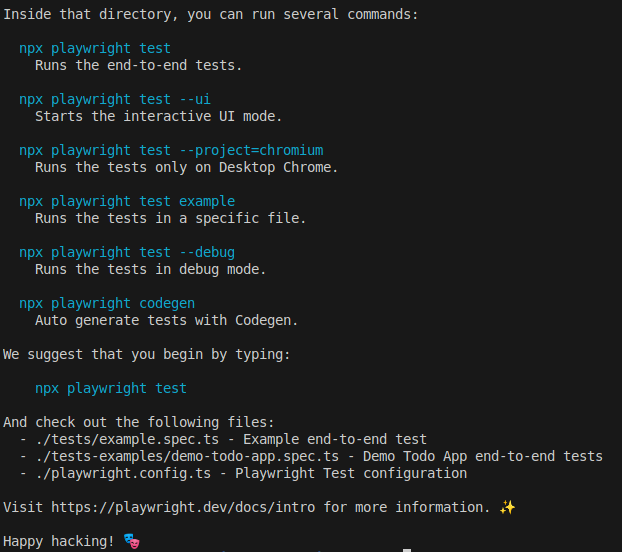
Theory
Playwright allows you to listen to various page events. One of them, console
, enables you to capture ConsoleMessage
objects. Each console message triggers an event.
You can retrieve:
- The type
- The message
- The origin of the message (file, line, and column)
- The originating page
Here’s a basic implementation:
|
|
Implementation
Now that we have the theory, let’s apply it to our HTML files.
We’ll create a scenario with three steps:
- Before each test:
- Listen to the
console
event and capture errors and warnings.
- Listen to the
- After each test:
- Reset the errors and warnings on the page.
- For each test:
- Visit each HTML file.
- Verify the expected number of console errors and warnings.
Here’s the code:
|
|
And to run the tests, use this command:
|
|
Here’s the result:
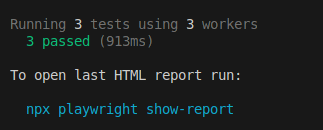